Buffers
A buffer is basically a space within the system memory that is used to store small packets of data for just about anything. Since it is held in system memory, it is very fast to access. You can think of it as a temporary place to put things that need to be worked on or processed.
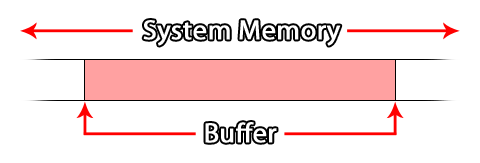
You can save and load ANY file into a buffer in SAMMI, which is the best part about having buffer commands!
For example, SAMMI does not natively support text files, but that’s okay, because you can simply load, read and save any text file using buffers instead!
Data Types
Buffer Type | Description |
---|---|
Unsigned 8bits | A single byte value. This is a positive value from 0 to 255. |
Signed 8bits | A single byte value. This can be a positive or negative value from -128 to 127 (0 is classed as positive). |
Unsigned 16bits | 2 bytes value. This is a positive value from 0 to 65,535. |
Signed 16bits | 2 bytes value. This can be a positive or negative value from -32,768 to 32,767 (0 is classed as positive). |
Unsigned 32bits | 4 bytes value. This is a positive value from 0 to 4,294,967,295. |
Signed 32bits | 4 bytes value. This can be a positive or negative value from -2,147,483,648 to 2,147,483,647 (0 is classed as positive). |
Float 32bits | 4 bytes value. This can be a positive or negative value from -2,147,483,648 to 2,147,483,647 that can contain decimal point numbers. |
Float 64bits | 8 bytes value. This can be a positive or negative value from 1-2^63 and 2^63 that can contain decimal point numbers. |
Boolean | A boolean value. Can only be either 1 or 0 (true or false). |
String | A string (text), finalized with a null terminating character. |
Text | A string(text) without the final null terminating character. Cannot be used for Buffer: Peak or Read commands (as the the length of a string is found by searching for the (first) null.) |
Creates a new buffer with the given name.
When creating a buffer, you should always try to create it to a size that is appropriate to the type, with the general rule being that it should be created to accommodate the maximum size of data that it is to store.
Buffer types:
- Fixed - A buffer of a fixed size in bytes. The size is set when the buffer is created and cannot be changed again. SAMMI will crash when you reach the limit size.
- buffExpand With Grow - A buffer that will grow dynamically as data is added. You create it with an initial size (which should be an approximation of the size of the data expected to be stored), and then it will expand to accept further data that overflows this initial size.
- Wrap - A buffer where the data will wrap. When the data being added reaches the limit of the buffer size, the overwrite will be placed back at the start of the buffer, and further writing will continue from that point.
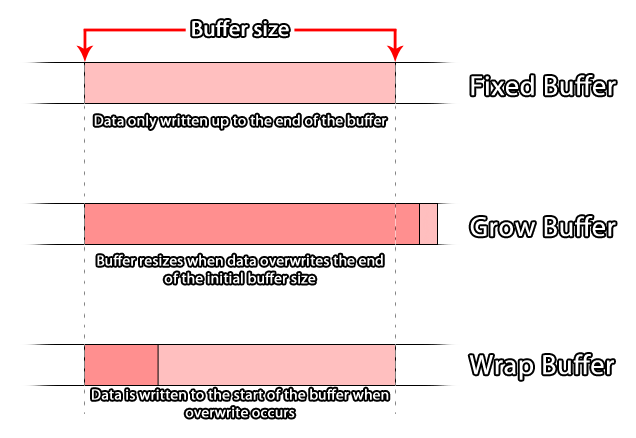
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer to create |
Byte Size | Size of the buffer in bytes. Default is 64. | |
Type | dropdown menu | Fixed, Expand With Grow or Wrap |
Writes data to a buffer at the specified position.
Writing data to a buffer will advance the position of the head by however many bytes it writes.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Type | Dropdown | Type of the buffer |
Value to Write | String | Data to write into the buffer |
Position | Int | Position to write the data at |
Writes data to a buffer at the position of the read/write head.
Writing data to a buffer will advance the position of the head by however many bytes it wrote.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Type | Dropdown | Type of the buffer |
Value to Write | String | Data to write into the buffer. |
Reads data from a buffer at the specified position.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Type | Drodown | Type of the buffer you want to read from |
Variable | String | Variable to save the read data into |
Position | Int | Position to read the data at |
Reads data from a buffer at the position of the read/write head.
Reading data from a buffer will advance the position of the head by however many bytes it reads.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Type | Drodown | Type of the buffer you want to read from |
Variable | String | Variable to save the read data into |
Loads any file as a buffer into SAMMI.
Read/Write head position will be set to 0 (beginning of the file).
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
File Path | String | File name to load. Accepts both relative and absolute path. |
With this command you can save the contents (raw data) of a buffer to a file, ready to be read back into memory using the Buffer: Load command. If a folder has not been created, a new one will be created with this command.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
File Path | String | File name to save the buffer into. Accepts both relative and absolute path. |
Example File Path: /$global.main_directory$/test.txt
Deletes a buffer you created or loaded, releasing the resources used to create it and removing any data that it may currently contain.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer to delete |
Changes the size of a buffer to a new size, for example to prevent overflow.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
New Size | Int | New size of the buffer. Default is 64. |
Sets the position of the read/write head of a buffer.
When you write or read from a buffer, data is not reshuffled. Rather, the head pointer is adjusted.
Whenever you use Buffer: Write command, head pointer advances. Whenever you use Buffer: Read command, head pointer also advances.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Position | Int | Value to set the position of the head to. |
Returns the size of a buffer.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Save Variable As | String | Variable to save the size value in |
Returns the position of a buffer’s read/write head.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Save Variable As | String | Variable to save the position value in |
Checks if a buffer exists and saves the result in a variable.\
Result:
- 0 - Buffer doesn’t exist
- 1 - Buffer exists
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Save Variable As | String | Variable to save the result in. |
Returns the SHA1 hash from a buffer. The data will be analyzed from 0 to the size of the buffer.
SHA1 hash is like a file’s digital signature. You can verify a file’s integrity by checking its hash value. If even one byte in the file changes (i.e. the file is modified in any way), its hash value will be different.
Very useful command to periodically detect for any file changes.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Variable | String | Variable to save the SHA1 string in |
Turns a whole buffer into a base64 string.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
Start Position | Where to start the string encoding (to encode full buffer, input 0 ) |
|
Size | Size of the buffer (leave at 0 for current buffer size) |
|
Save Variable As | String | Variable to save the string value in |
Creates a new buffer from a base64 string.
Box Name | Type | Description |
---|---|---|
Buffer Name | String | Name of the buffer |
String (text) | String | A base64 string to decode and turn into a buffer. |